I made a video explaining how to do Whatsapp automation using Javascript. Someone has asked this question in the comments of that video. Let’s find out!
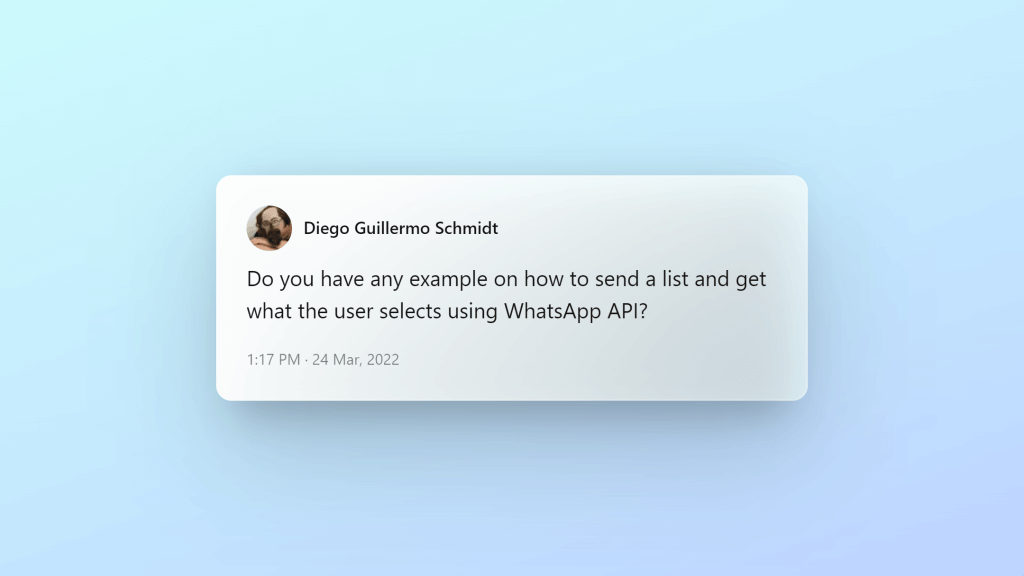
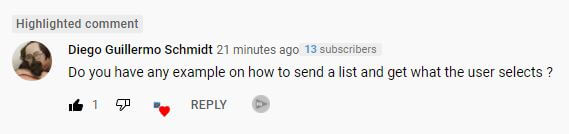
Here’s the example source code to send a list of products in which users can select one product. After selecting a product, an automatic message will be sent with the message “You’ve select X product”
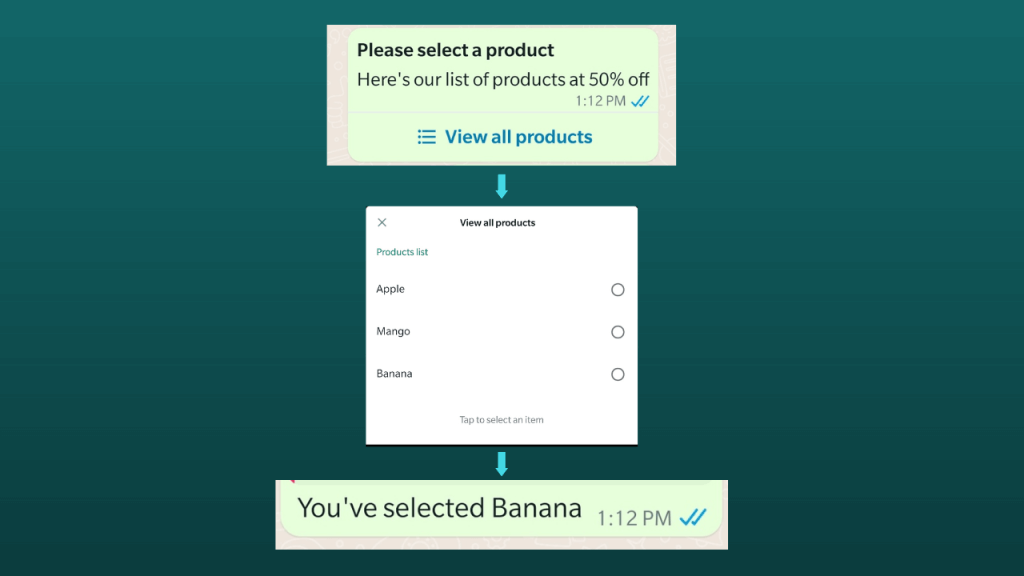
I’m sending the list to a group named “Source Group” in this example. You can change the group name or modify the code to send it to a contact based on your requirement.
const { Client, LocalAuth, Buttons, List } = require("whatsapp-web.js");
const qrcode = require("qrcode-terminal");
const myGroupName = "Source Group";
const client = new Client({
authStrategy: new LocalAuth(),
});
client.on("qr", (qr) => {
qrcode.generate(qr, { small: true });
});
client.on("ready", () => {
console.log("Client is ready!");
client.getChats().then((chats) => {
myGroup = chats.find((chat) => chat.name === myGroupName);
const productsList = new List(
"Here's our list of products at 50% off",
"View all products",
[
{
title: "Products list",
rows: [
{ id: "apple", title: "Apple" },
{ id: "mango", title: "Mango" },
{ id: "banana", title: "Banana" },
],
},
],
"Please select a product"
);
client.sendMessage(myGroup.id._serialized, productsList);
});
});
client.on("message", (message) => {
if(message.type === 'list_response'){
message.reply(`You've selected ${message.body}`);
}
});
client.initialize();
Latest posts by Ranjith kumar (see all)
- Ultimate Guide: Build A Mobile E-commerce App With React Native And Medusa.js - February 15, 2025
- Flutter lookup failed in @fields error (solved) - July 14, 2023
- Free open source alternative to Notion along with AI - July 13, 2023