Removing background from an Image is something we need quite often. We’ll be searching for background removal tools. Here’s how to remove background programmatically using Javascript.
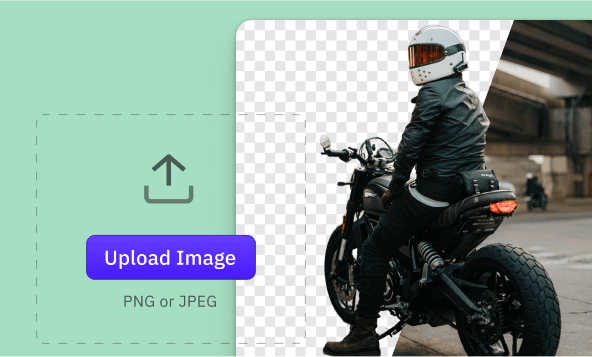
Introducing background-removal-js
Remove backgrounds from images directly in the browser environment with ease and no additional costs or privacy concerns.
Overview
@imgly/background-removal
is a powerful npm package that allows developers to seamlessly remove the background from images directly in the browser. With its unique features and capabilities, this package offers an innovative and cost-effective solution for background removal tasks without compromising data privacy.
Key features
The key features of @imgly/background-removal
are:
- In-Browser Background Removal: Our one-of-a-kind solution performs the entire background removal process directly in the user’s browser, eliminating the need for additional server costs. By leveraging the computing power of the local device, users can enjoy a fast and efficient background removal process.
- Data Protection: As
@imgly/background-removal
runs entirely in the browser, users can have peace of mind knowing that their images and sensitive information remain secure within their own devices. With no data transfers to external servers, data privacy concerns are effectively mitigated. - Seamless Integration with IMG.LY’s CE.SDK:
@imgly/background-removal
provides seamless integration with IMG.LY’s CE.SDK, allowing developers to easily incorporate powerful in-browser image matting and background removal capabilities into their projects.
The Neural Network (ONNX model) and WASM files used by @imgly/background-removal
are hosted on UNPKG, making it readily available for download to all users of the library. See the section Custom Asset Serving if you want to host data on your own servers.
Installation
You can install @imgly/background-removal
via npm or yarn. Use the following commands to install the package:
npm install @imgly/background-removal
Usage
import imglyRemoveBackground from "@imgly/background-removal"
let image_src: ImageData | ArrayBuffer | Uint8Array | Blob | URL | string = ...;
imglyRemoveBackground(image_src).then((blob: Blob) => {
// The result is a blob encoded as PNG. It can be converted to an URL to be used as HTMLImage.src
const url = URL.createObjectURL(blob);
})
Note: On the first run the wasm and onnx model files are fetched. This might, depending on the bandwidth, take time. Therefore, the first run takes proportionally longer than each consecutive run. Also, all files are cached by the browser and an additional model cache.
Advanced Configuration
The library does not need any configuration to get started. However, there are optional parameters that influence the behavior and give more control over the library.
type Config = {
publicPath: string; // The public path used for model and wasm files
debug: bool; // enable or disable useful console.log outputs
proxyToWorker: bool; // Whether to proxy the calculations to a web worker. (Default true)
model: 'small' | 'medium'; // The model to use. (Default "medium")
};
Download Size vs Quality
The onnx model is shipped in various sizes and needs.
- small (~40 MB) is the smallest model and is in most cases working fine but sometimes shows some artifacts. It’s a quantized model.
- medium (~80MB) is the default model.
Download Progress Monitoring
On the first run, the necessary data will be fetched and stored in the browser cache. Since the download might take some time, you have the option to tap into the download progress.
let config: Config = {
progress: (key, current, total) => {
console.log(`Downloading ${key}: ${(current} of ${total}`);
}
}
Who is it for?
is ideal for developers and projects that require efficient and cost-effective background removal directly in the browser. It caters to a wide range of use cases, including but not limited to:@imgly/background-removal
- E-commerce applications that need to remove backgrounds from product images in real time.
- Image editing applications that require background removal capabilities for enhancing user experience.
- Web-based graphic design tools that aim to simplify the creative process with in-browser background removal.
Whether you are a professional developer or a hobbyist, @imgly/background-removal
empowers you to deliver impressive applications and services with ease.
Demo of image with background removed
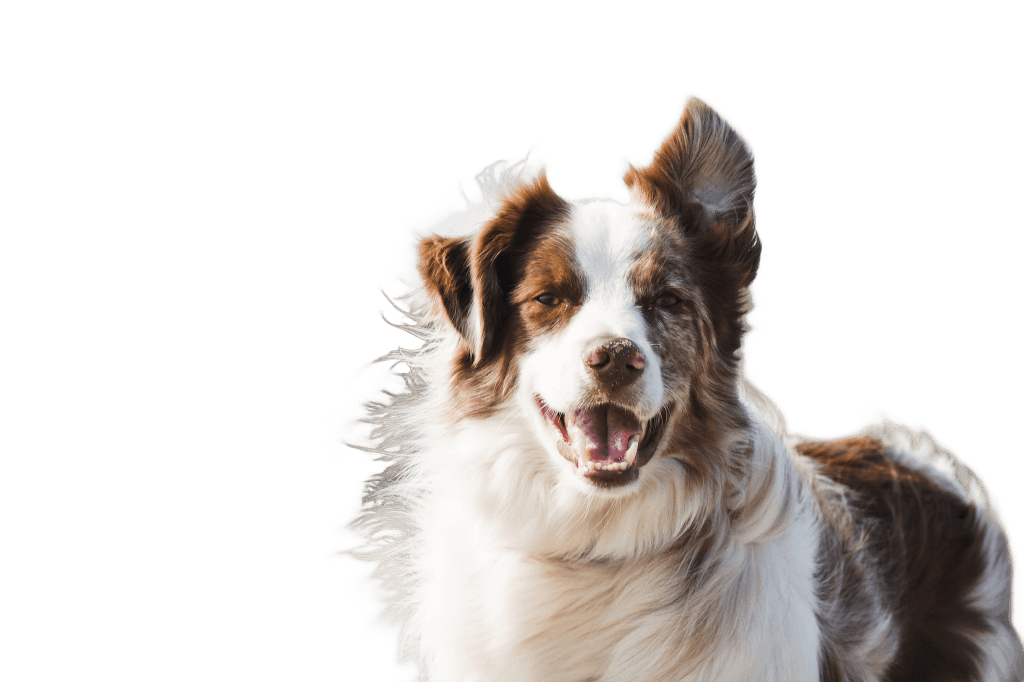
Live Demo
A live demo of the background removal tool in Javascript can be explored here
- Ultimate Guide: Build A Mobile E-commerce App With React Native And Medusa.js - February 15, 2025
- Flutter lookup failed in @fields error (solved) - July 14, 2023
- Free open source alternative to Notion along with AI - July 13, 2023
Very useful practical implementation of javascript.