When designing a web application or website, it’s common to include functionality that prompts users with a confirmation dialog before they leave or close the page. This feature is useful to prevent users from accidentally navigating away and losing unsaved changes.
In this blog post, we’ll explore how to implement a confirmation dialog using JavaScript’s beforeunload
event.
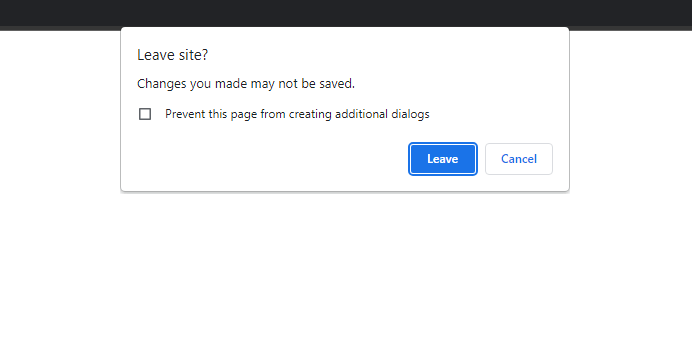
The beforeunload Event
The beforeunload
event is triggered when a user attempts to leave a page. By adding an event listener to this event, we can execute a function that displays a confirmation dialog before allowing the user to proceed.
Usage
We can use the beforeunload Event in 2 ways to show a confirmation dialog to user before leaving the page.
addEventListener method
window.addEventListener('beforeunload', function (event) {
event.preventDefault();
return (event.returnValue = "");
});
onbeforeunload property in HTML
<body onbeforeunload="return myFunction()">
<h1>The beforeunload Event</h1>
<p>Reload this page, or click on the link below to invoke the onbeforeunload event.</p>
<a href="https://codingislove.com">Click here to go to codingislove.com</a>
<script>
function myFunction() {
event.preventDefault();
return "";
}
</script>
</body>
Demo
Here’s a live demo below
Practical use case
Let’s say the user is filling out a form and he tries to exit the page, then we can show him a confirmation dialog that he has unsaved changes and get confirmation to proceed.
Try to use this event only for reasonable use cases to avoid any performance issues.
Unable to customize the message shown in onbeforeload?
If you are not able to change the confirmation message of onbeforeload event, that is because Starting in Chrome 51, a custom string will no longer be shown to the user. Chrome will still show a dialog to prevent users from losing data, but it’s contents will be set by the browser instead of the web page.
Chrome stopped support custom message in onbeforeload event from Chrome 51 because the developers were misusing this feature to scam the users! 😂
Browser Limitations
t’s important to note that different browsers may impose limitations on the customization of the confirmation dialog to prevent abuse or security issues. As a result, the appearance and behavior of the dialog may vary across platforms and browser versions.
Conclusion
We hope this blog post has provided you with a clear understanding of how to add a confirmation dialog before closing a web page. Happy coding ✌️
- Flutter lookup failed in @fields error (solved) - July 14, 2023
- Free open source alternative to Notion along with AI - July 13, 2023
- Threads API for developers for programmatic access - July 12, 2023