Threads, An Instagram app is all the rage now. Threads is an alternative to Twitter and everyone’s hoping on the new platform now. If you’re a developer and looking for Threads API then continue reading.
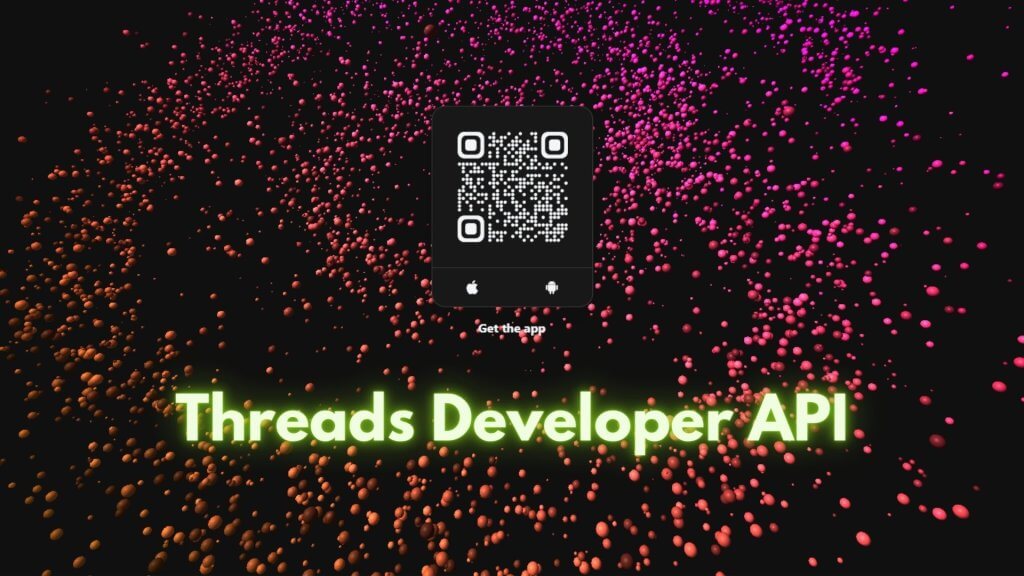
Threads API
There is no official developer API for Threads yet but a developer reverse-engineered the Threads API requests and created an unofficial API client.
The API client is an unofficial, Reverse-Engineered Node.js/TypeScript client for Meta’s Threads.
📦 Installation
yarn add threads-api
# or with npm
npm install threads-api
# or with pnpm
pnpm install threads-api
// or in Deno 🦖
import ThreadsAPI from 'npm:threads-api';
const threadsAPI = new ThreadsAPI.ThreadsAPI({});
Threads API Usage
It is as simple as importing the API client and using the client’s methods. If you want to access public data then you can directly use the API but if you want to access private methods such as publishing a thread or following someone then you should initialize the API with username and password
Checkout the examples below:
How to fetch threads user profile from API
import APIClient from "threads-api";
const main = async () => {
const threadsAPI = new APIClient.ThreadsAPI();
const username = "codingislove";
// 👤 Details for a specific user
const userID = await threadsAPI.getUserIDfromUsername(username);
if (!userID) {
return;
}
const user = await threadsAPI.getUserProfile(username, userID);
console.log(JSON.stringify(user));
};
main();
Response:
{
"is_private": false,
"profile_pic_url": "https://scontent.cdninstagram.com/v/t51.2885-19/358193075_291108399947602_86677529474390528_n.jpg?stp=dst-jpg_s150x150&_nc_ht=scontent.cdninstagram.com&_nc_cat=110&_nc_ohc=qa3mujfMuOMAX_hdows&edm=APs17CUBAAAA&ccb=7-5&oh=00_AfBjrANoN_vgzct9EOFSe3NZACDpJijVQbwXnUsgUIkDuQ&oe=64B34485&_nc_sid=10d13b",
"username": "codingislove",
"hd_profile_pic_versions": null,
"is_verified": false,
"biography": "If you're a developer, you should follow this account 🤖",
"biography_with_entities": null,
"follower_count": 4,
"profile_context_facepile_users": null,
"bio_links": [{ "url": "https://codingislove.com/" }],
"pk": "5635301639",
"full_name": "Coding is Love",
"id": null
}
✨ How to publish a thread using API
import APIClient from "threads-api";
const publishThread = async () => {
const threadsAPI = new APIClient.ThreadsAPI({
username: '_junhoyeo', // Your username
password: 'PASSWORD', // Your password
});
await threadsAPI.publish({
text: '🤖 Hello World',
});
};
publishThread();
✨ Threads with Image
await threadsAPI.publish({
text: '🤖 Threads with Image',
image: 'https://github.com/junhoyeo/threads-api/raw/main/.github/cover.jpg',
});
✨ Threads with Link Attachment
await threadsAPI.publish({
text: '🤖 Threads with Link Attachment',
url: 'https://github.com/junhoyeo/threads-api',
});
✨ Reply to Other Threads
const parentURL = 'https://www.threads.net/t/CugF-EjhQ3r';
const parentPostID = await threadsAPI.getPostIDfromURL(parentURL); // or use `getPostIDfromThreadID`
await threadsAPI.publish({
text: '🤖 Beep',
link: 'https://github.com/junhoyeo/threads-api',
parentPostID: parentPostID,
});
✨ Like/Unlike a Thread
const threadURL = 'https://www.threads.net/t/CugK35fh6u2';
const postIDToLike = await threadsAPI.getPostIDfromURL(threadURL); // or use `getPostIDfromThreadID`
// 💡 Uses current credentials
await threadsAPI.like(postIDToLike);
await threadsAPI.unlike(postIDToLike);
✨ Follow/Unfollow a User
const userIDToFollow = await threadsAPI.getUserIDfromUsername('junhoyeo');
// 💡 Uses current credentials
await threadsAPI.follow(userIDToFollow);
await threadsAPI.unfollow(userIDToFollow);
❤️🔥 Delete a Post
const postID = await threadsAPI.publish({
text: '🤖 This message will self-destruct in 5 seconds.',
});
await new Promise((resolve) => setTimeout(resolve, 5_000));
await threadsAPI.delete(postID);
Supported features
- ✅ Read public data
- ✅ Fetch UserID(
314216
) via username(zuck
) - ✅ Read User Profile Info
- ✅ Read the list of User Threads
- ✅ Read the list of User Replies
- ✅ Fetch PostID(
3140957200974444958
) via PostID(CuW6-7KyXme
) or PostURL(https://www.threads.net/t/CuW6-7KyXme
) - ✅ Read Threads via PostID
- ✅ Read Likers in Thread via PostID
- 🚧 Read User Followers
- 🚧 Read User Followings
- ✅ Fetch UserID(
- 🚧 Read private data
- ✅ Write data (i.e. write automated Threads)
- ✅ Create a new Thread with text
- ✅ Make link previews to get shown
- ✅ Create a new Thread with a single image
- 🚧 Create a new Thread with multiple images
- ✅ Reply to existing Thread
- ✅ Delete Thread
- ✅ Create a new Thread with text
- ✅ Friendships
- ✅ Follow User
- ✅ Unfollow User
- ✅ Interactions
- ✅ Like Thread
- ✅ Unlike Thread
Conclusion
Checkout the official repo for more options and updates
Also, read How to Send SMS from API or Backend 📲
- Flutter lookup failed in @fields error (solved) - July 14, 2023
- Free open source alternative to Notion along with AI - July 13, 2023
- Threads API for developers for programmatic access - July 12, 2023