I made a video explaining how to do Whatsapp automation using Javascript. Someone has asked this question in the comments of that video asking about how to add participants to a WhatsApp group using whatsapp-web.js Let’s find out!
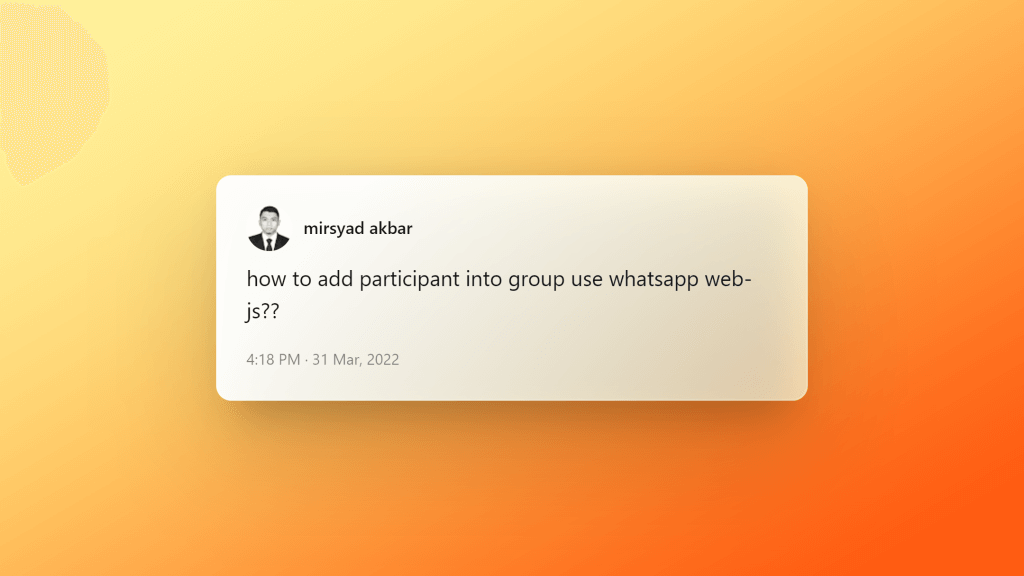

Example source code
Here’s the example source code to add participants to a group using Whatsapp API
const { Client, LocalAuth } = require("whatsapp-web.js");
const qrcode = require("qrcode-terminal");
const myGroupName = "Group Name";
const contactName = "John Doe";
const client = new Client({
authStrategy: new LocalAuth(),
});
client.on("qr", (qr) => {
qrcode.generate(qr, { small: true });
});
client.on("ready", () => {
console.log("Client is ready!");
client.getChats().then((chats) => {
const myGroup = chats.find((chat) => chat.name === myGroupName);
client.getContacts().then((contacts) => {
const contactToAdd = contacts.find(
// Finding the contact Id using the contact's name
(contact) => contact.name === contactName
);
if (contactToAdd) {
myGroup
.addParticipants([contactToAdd.id._serialized]) // Pass an array of contact IDs [id1, id2, id3 .....]
.then(() =>
console.log(
`Successfully added ${contactName} to the group ${myGroupName}`
)
);
} else {
console.log("Contact not found");
}
});
});
});
client.initialize();
Adding participants using phone number
All we need to do is pass a list of contact IDs to addParticipants
method.
In this example, I’m finding the contact ID using the contact’s name but you can directly pass the contact ID if you already have the phone number of the contact.
contact ID will be in this format – 919XXXXXXXXX@c.us
91 is the country code of India. Replace it with your country code. Replace the remaining part with the actual phone number. @c.us
remains the same for all contacts in all countries.
If you are passing IDs directly then the code would look something like this:
myGroup.addParticipants(["[email protected]", "[email protected]"])
- Flutter lookup failed in @fields error (solved) - July 14, 2023
- Free open source alternative to Notion along with AI - July 13, 2023
- Threads API for developers for programmatic access - July 12, 2023
Please show me how to get group info like group name, group description using whatsapp web js
Hi, You can see that in the above example. chat.name is nothing but group name in this case.
How can I add one contact ID to more than one group at the same time?
Hi, You can use a for loop
Please can you provide an example
i want samething but don’t know java would like to do in excel vba kindly provide code for the same
I wrote the code but the code fails to add people who are not in your contact list. The code can only add people who are in your contact list
That is probably a security limitation set by Whatsapp
yeah apparently, I was looking for ways to add people to group without having to add their contact number. Now I guess I am left with no other option than to send an invite link.
Hi, getContacts() not getting all of my contacts. I am unable to add people to my group who are in my contact. Kindly help me.
Hi, I getContacts() not returning all of my contacts. So I couldn’t add people to my group who are in my contact. Kindly help me.
Hi, Can you tell me which contacts are returning and which contacts are not returning?
I have above 1000 contacts but it is returning only 10.
Um, not sure what’s the problem. I’m getting all the contacts. You can make sure that you are using the latest version of the library.
I am using the latest library “whatsapp-web.js”: “^1.21.0”.
Sometimes it is working fine. Sometimes it is not.
And It is returning that 10 contacts name as undefined.
PrivateContact {
id: {
server: ‘c.us’,
user: ’91XXXXXXX’,
_serialized: ’[email protected]’
},
number: undefined,
isBusiness: false,
isEnterprise: false,
labels: undefined,
name: undefined,
pushname: undefined,
sectionHeader: undefined,
shortName: undefined,
statusMute: undefined,
type: ‘in’,
verifiedLevel: undefined,
verifiedName: undefined,
isMe: undefined,
isUser: undefined,
isGroup: undefined,
isWAContact: undefined,
isMyContact: undefined,
isBlocked: false
},
Hi, Can I send a message to the group? I am trying to send a message to my group. But nothing happens.
await client.sendMessage(
</strong><span style="color: rgb(86, 156, 214);">${</span><span style="color: rgb(79, 193, 255);">myGroup</span>.<span style="color: rgb(156, 220, 254);">groupMetadata</span>.<span style="color: rgb(156, 220, 254);">id</span>.<span style="color: rgb(156, 220, 254);">user</span><span style="color: rgb(86, 156, 214);">}</span><span style="color: rgb(206, 145, 120);">@g.us</span><strong>
,message);I am using this code. Kindly help me.
You seem to using wrong code, check my other articles on how to send a message to a whatsapp group
Sorry, my mistake. I am using this code.
await client.sendMessage(‘[email protected]’,message);
Can you share the article plz?