Allowing a user to copy text by clicking a button is a common pattern in websites. Let’s see how to copy text to clipboard using Javascript
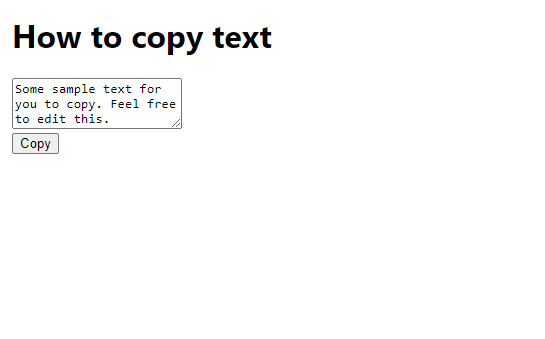
Copy using the navigator.clipboard API
Here’s how to copy or set some text to the clipboard:
navigator.clipboard.writeText("Some text to be copied");
Full source code
Here’s the full source code and live demo
HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<link
rel="icon"
href="data:image/svg+xml,<svg xmlns=%22http://www.w3.org/2000/svg%22 viewBox=%220 0 100 100%22><text y=%22.9em%22 font-size=%2290%22>🎉</text></svg>"
/>
<title>How to copy text</title>
</head>
<body>
<h1>How to copy text</h1>
<textarea rows="3">
Some sample text for you to copy. Feel free to edit this.</textarea
>
<button type="button">Copy</button>
</body>
</html>
JS:
const textarea = document.querySelector('textarea');
const button = document.querySelector('button');
button.addEventListener('click', async () => {
try {
await navigator.clipboard.writeText(textarea.value);
} catch (err) {
console.error(err.name, err.message);
}
});
Live Demo
See the Pen Copy text to clipboard by Ranjith (@Ranjithkumar10) on CodePen.
Latest posts by Ranjith kumar (see all)
- Flutter lookup failed in @fields error (solved) - July 14, 2023
- Free open source alternative to Notion along with AI - July 13, 2023
- Threads API for developers for programmatic access - July 12, 2023